Unlocking the Power of Functional Programming in R (Part 1)
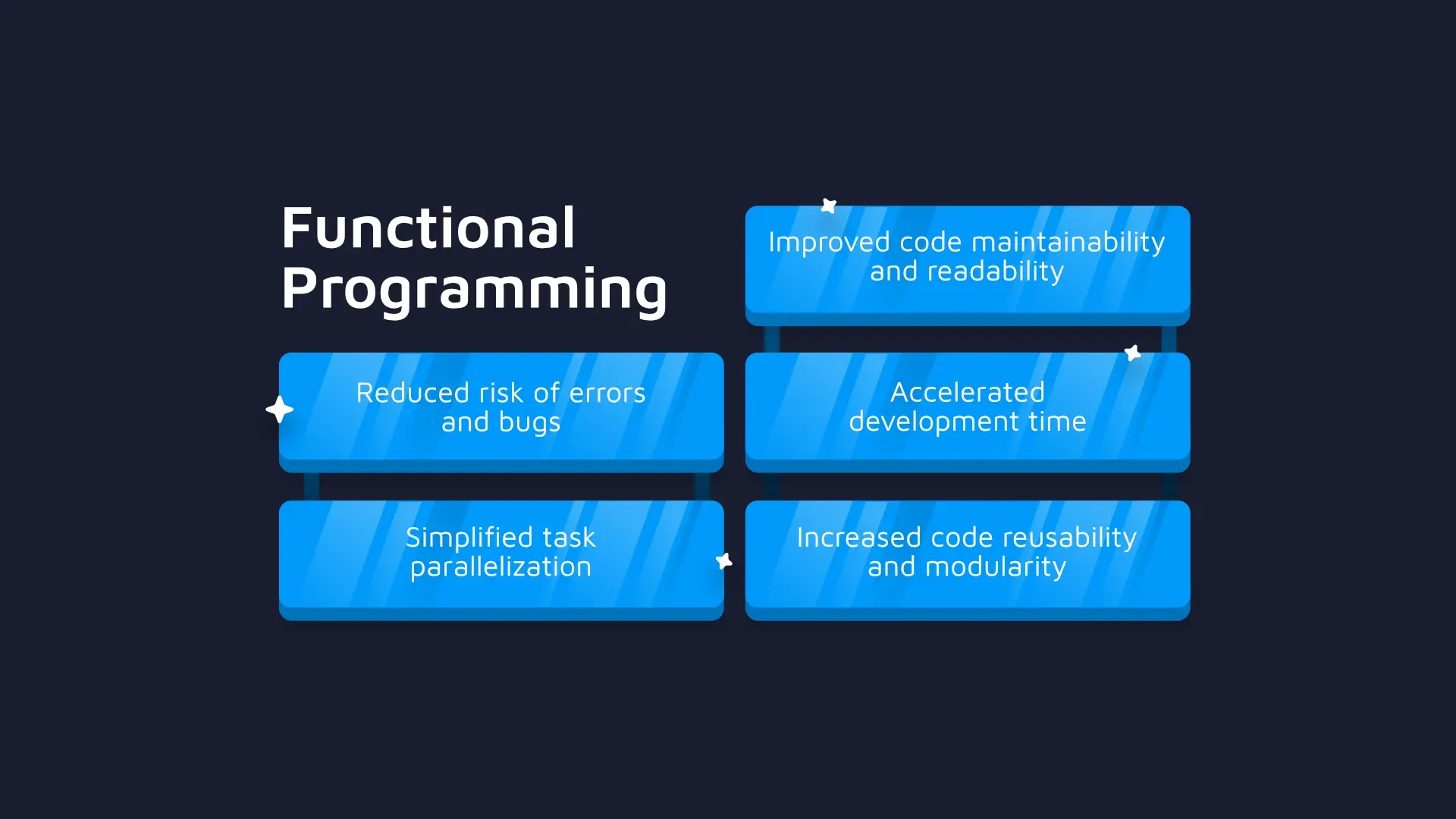
In the ever-evolving landscape of software development, there exists a paradigm that has been <a href="https://academic.oup.com/nsr/article/2/3/349/1427872?login=false">gaining momentum</a> and reshaping the way we approach coding challenges: functional programming. In this article, we delve deep into the world of functional programming, exploring its advantages, core principles, origin, and reasons behind its growing traction. This is the first of a multiple-part series where we will delve into the intricacies of functional programming in R. <h3>TL;DR:</h3><ul><li>Functional programming revolves around <strong>functions</strong> as <strong>primary building blocks</strong>.</li><li>It emphasizes <strong>pure functions</strong>, <strong>immutability</strong>, <strong>higher-order functions</strong>, and <strong>declarative style</strong>.</li><li>It offers the following benefits:<ul><li>Accelerated development time</li><li>Improved code maintainability and readability</li><li>Reduced risk of errors and bugs</li><li>Increased code reusability and modularity</li><li>Scalability and performance</li><li>Simplified task parallelization</li></ul> </li> </ul> <h3><strong>Table of Contents</strong></h3><ul><li><a href="#functional-programming">What is Functional Programming?</a></li><li><a href="#why-choose">Why Choose Functional Programming?</a></li><li><a href="#understanding-functional">Understanding Functional Programming</a></li></ul> <hr /> <h2 id="functional-programming">What is Functional Programming?</h2> <strong>Functional programming (FP)</strong> is a programming paradigm that offers a unique approach to data processing workflows. As the name suggests, functional programming revolves around the concept of functions. In contrast to both traditional imperative programming, where code follows sequences of state-changing instructions, and object-oriented programming, which organizes code around objects and their interactions, functional programming treats functions as the main constructs for data manipulation and analysis. <h3>Elevating Functions to First-Class Citizens</h3> Functions are first-class citizens in functional programming, which means they can be treated like any other data type. You can assign functions to immutable constants, pass them as arguments to other functions, and return them as results from functions. This flexibility enables a powerful level of abstraction and modularity in the code. <a href="https://share.hsforms.com/1J9uLL_NdSdW4wADt50b1Vw2rk4g?utm_source=website&utm_medium=blog&utm_campaign=fp1" target="_blank" rel="noopener"><img class="aligncenter wp-image-22433 size-full" src="https://webflow-prod-assets.s3.amazonaws.com/6525256482c9e9a06c7a9d3c%2F65b00c6e83526ad59a7ab3ee_Artboard1_6.webp" alt="functional programming ebook" width="1045" height="383" /></a> <h2 id="why-choose">Why Choose Functional Programming?</h2> Functional programming meets the evolving demands of modern data science. Its principles and methodologies provide robust solutions, aligning seamlessly with technical and business needs in data-driven industries. On the technical side of things, these factors include: <ul><li><strong><em>Promoting the use of pure functions</em></strong>, which have no side effects and produce predictable outputs for given inputs. This predictability enhances code reliability and makes it easier to reason about complex systems, which is crucial in today's software development landscape, where scalability and maintainability are paramount.</li><li><em><strong>Functional programming languages and paradigms encourage immutability</strong></em>, meaning that once data is defined, it cannot be changed. This immutability helps prevent bugs that can arise from unintended data modifications, leading to more robust and bug-free software. Also, immutability makes it easier to parallelize code execution, a critical requirement for modern software systems that need to leverage multi-core processors effectively.</li><li><em><strong>Functional programming languages often include powerful features like higher-order functions, pattern matching, and type inference</strong></em>, which facilitate code abstraction and reuse. These features contribute to shorter development cycles, faster time-to-market, and reduced development costs.</li><li><em><strong>Functional programming simplifies parallelizing tasks</strong></em>. Leveraging its statelessness, operations like loops can be concurrently executed, optimizing computations. This ensures both swift data processing and optimizing use of computational resources, which is essential in today’s data-intensive landscape.</li></ul> Here’s how functional programming subtly intertwines with business value: <ul><li><em><strong>Functional Programming is crucial for organizations aiming at cost-efficient software development</strong></em>, offering a robust framework that ensures data integrity and functional reliability. This approach leads to a dependable codebase, reducing both time and financial resources allocated for debugging, which is a critical factor for economical project execution.</li><li><em><strong>Time is a crucial resource in the software development lifecycle.</strong></em> The expressiveness of functional programming facilitates clear communication of developers' intentions through code, promoting efficient and accelerated development cycles. This results in better testing and easier refactoring, which can speed up product release timelines.</li><li><em><strong>Functional programming mitigates risks associated with software irregularities and operational interruptions</strong></em> by offering a predictable coding structure through modularity and composability. This structure not only reduces bug occurrences but also simplifies refactoring, thereby minimizing risks associated with software malfunctions.</li></ul> <h3>Languages Championing Functional Programming</h3> Languages such as R, Haskell, Scala, and Clojure offer concise and expressive syntax for handling common programming tasks. This brevity reduces code verbosity and minimizes the potential for bugs, resulting in more efficient and maintainable codebases. <img class="size-full wp-image-21195" src="https://webflow-prod-assets.s3.amazonaws.com/6525256482c9e9a06c7a9d3c%2F65b01a123bb3b9ef8e3c9d47_photo_2023-10-13_14-13-25.webp" alt="" width="708" height="578" /> Map and Filter Functional programming in R leverages functions as first-class citizens, facilitating modular and reusable code and enhancing the maintainability of complex data analysis workflows. Also, it encourages immutability, reducing the chances of unintended side effects in data processing, which is vital in statistical computing where data integrity is paramount. The combination of functional programming principles with R's data-centric capabilities makes it a powerful choice for data-driven applications, data science projects, and statistical modeling. This unique approach not only accelerates the development cycles but also significantly trims down the associated costs, reduces bugs and errors, resulting in considerably less time and financial resources spent on debugging and fixing while providing a smooth, hassle-free development experience. This synergy between functional programming and R opens up new possibilities for extracting meaningful insights from data and meeting the demands of today's data-driven industries. Although we are introducing the concept of functional programming in this part of the series, we will delve into the specifics of applying these principles in R in subsequent parts. <h2 id="understanding-functional">Understanding Functional Programming</h2> <h3>Core Principles</h3> Functional programming is characterized by several key principles that distinguish it from traditional imperative programming: <h4>Pure Functions</h4> In functional programming, functions are pure, meaning they consistently produce the same output for the same input, without any side effects. This predictability makes code more reliable and easier to test. To aid in easier grasp, our examples will be demonstrated using Python. <pre><code> # Pure Function Example def add(a, b): return a + b <br>result = add(3, 5) # Calling the pure function print(result) # Output: 8 <br># Impure Function Example (with side effect) total = 0 <br>def impure_add(a, b): global total # Modifying external state (side effect) total += a + b return total <br>result = impure_add(3, 5) # Calling the impure function print(result) # Output: 8 <br>result = impure_add(2, 5) # Calling the impure function print(result) # Output: 15 ( Incorrect result ) </code></pre> In the example, add(a, b) is a pure function because it takes two arguments, a and b, and it always returns the same result for the same inputs without modifying any external state or variables. This predictability and lack of side effects make it a pure function. On the other hand, impure_add(a, b) is an impure function because it not only calculates the result but also modifies the global variable total, which is a side effect. This impurity can make code less reliable and harder to test compared to pure functions in functional programming. <h4>Immutability</h4> Instead of modifying existing data, FP promotes creating new data structures, which are never changed once created. This approach simplifies reasoning about data and helps prevent bugs caused by unintended alterations. <pre><code> # Mutable Data (Non-functional approach) my_list = [1, 2, 3] <br># Modifying the list my_list.append(4) my_list[0] = 10 <br>print(my_list) # Output: [10, 2, 3, 4] <br># Immutable Data (Functional approach) immutable_list = (1, 2, 3) <br># Creating a new tuple with an additional element new_immutable_list = immutable_list + (4,) <br># Attempting to modify the original tuple (will result in an error) # immutable_list[0] = 10 # This line will raise a TypeError <br>print(new_immutable_list) # Output: (1, 2, 3, 4) </code></pre> In this example, we demonstrate immutability by using a Python tuple <code>immutable_list</code>. Instead of modifying the original tuple, we create a new one <code>new_immutable_list</code> by combining it with another tuple containing the additional element (4). Attempting to modify the original tuple results in a TypeError, highlighting the immutability aspect of functional programming, where data structures are never changed once created. This approach simplifies reasoning about data and helps prevent unintended alterations, leading to more predictable and maintainable code. <h4>Higher-Order Functions</h4> This allows functions to be passed as arguments to other functions or returned as results. This concept enables the creation of powerful abstractions and facilitates more modular & reusable code. <pre><code> # Higher-Order Function Example def apply_operation(operation, x, y): return operation(x, y) <br># Define some operations as functions def add(x, y): return x + y <br>def subtract(x, y): return x - y <br>def multiply(x, y): return x * y <br>def divide(x, y): if y != 0: return x / y else: return "Division by zero is not allowed." <br># Using the higher-order function to perform various operations result1 = apply_operation(add, 5, 3) print("Addition:", result1) # Output: 8 <br>result2 = apply_operation(subtract, 10, 4) print("Subtraction:", result2) # Output: 6 <br>result3 = apply_operation(multiply, 6, 2) print("Multiplication:", result3) # Output: 12 <br>result4 = apply_operation(divide, 8, 2) print("Division:", result4) # Output: 4.0 </code></pre> In this example, apply_operation is a higher-order function that takes three arguments: operation, x, and y. The operation argument is a function itself, which can be any of the arithmetic operations defined (add, subtract, multiply, or divide). The apply_operation function applies the specified operation to x and y, allowing you to perform different operations by passing different functions as arguments. This demonstrates how higher-order functions enable the creation of powerful abstractions and facilitate modular and reusable code, as you can easily switch and combine operations as needed. <h4>Declarative Programming</h4> Functional programming encourages a declarative style, where you specify what you want to achieve rather than detailing step-by-step instructions for how to achieve it. This results in more concise and readable code. <pre><code> # Imperative Approach (Non-declarative) numbers = [1, 2, 3, 4, 5] doubled_numbers = [] <br>for num in numbers: doubled_numbers.append(num * 2) <br>print("Doubled Numbers (Imperative):", doubled_numbers) <br># Declarative Approach numbers = [1, 2, 3, 4, 5] doubled_numbers = list(map(lambda x: x * 2, numbers)) <br>print("Doubled Numbers (Declarative):", doubled_numbers) </code></pre> In the imperative approach, we use a for loop to iterate over a list of numbers and manually append the doubled values to a new list <code>doubled_numbers</code>. This approach involves specifying step-by-step instructions on how to achieve the desired result. In contrast, the declarative approach uses the map function, which takes a lambda function to define the doubling operation and applies it to each element in the numbers list. This approach specifies what we want to achieve (doubling each number) rather than detailing the step-by-step process. It results in more concise and readable code, as the intent of the operation is clear without explicit iteration and temporary variables. <h4>Concurrency and Parallelism</h4> Immutable data and avoidance of shared state make it well-suited for building concurrent and parallel systems, crucial in industries like finance, telecommunications, and gaming. <h4>Data Processing</h4> Functional Programming excels in data transformation and analysis tasks, making it a natural fit for data science, big data, and analytics applications. <h4>Safety and Reliability</h4> The emphasis on purity and immutability reduces common programming errors, making functional programming appealing in industries where software reliability is critical, such as healthcare and aerospace. <h4>Scalability</h4> Lastly, it promotes modularity and code composition, easing the development of scalable applications. <h3>Origin</h3> <h4>Lambda Calculus</h4> The foundational concept of functional programming finds its inception in <a href="https://en.wikipedia.org/wiki/Lambda_calculus">lambda calculus</a>, a formal system of mathematical logic invented by Alonzo Church in the 1930s. Church introduced lambda calculus as a means of exploring the foundations of computation and the nature of functions. It provided a formal notation for defining and applying functions, laying the groundwork for functional programming's core tenets. <h4>Lisp</h4> In the 1950s and 1960s, functional programming began to gain prominence in the emerging field of computer science. Notably, John McCarthy, widely recognized as one of the pioneers of artificial intelligence, played a pivotal role in this development. He introduced the Lisp programming language (short for "LISt Processing"), which incorporated lambda calculus concepts and became one of the earliest functional programming languages. Lisp's elegant support for symbolic computation and its emphasis on recursion and higher-order functions made it a groundbreaking language in the functional programming realm. Alonzo Church's work on the lambda calculus remained influential during this period as well. His mathematical formalism provided a theoretical underpinning for functional programming languages and their principles. Church's contributions not only shaped the theoretical foundation of functional programming but also inspired the development of practical languages. <pre><code> # Lisp code (defun factorial (n) (if (<= n 1) 1 (* n (factorial (- n 1))))) ; Calculate the factorial of 5 (format t "Factorial of 5: ~A" (factorial 5)) </code></pre> In this Lisp code, we define a function factorial that calculates the factorial of a number n using recursion. It checks if n is less than or equal to 1, and if so, returns 1 (the base case). Otherwise, it recursively calls itself with n decremented by 1 and multiplies the result by n. Finally, we calculate and print the factorial of 5 using this function. <h3>Recent Popularity</h3> The reasons for the growing traction of functional programming in recent years are multifaceted. <h4>Concurrency and Parallelism</h4> Given that multi-core processors have been the standard for the past 15 years, traditional imperative programming's shared-state and mutable data models have become more error-prone and challenging to manage in concurrent and parallel systems. Functional programming provides a more natural and safer way to handle concurrency with its emphasis on immutability and pure functions. This results in improved system performance and reliability, which are essential for businesses aiming to deliver high quality, responsive software. <img class="size-full wp-image-21197" src="https://webflow-prod-assets.s3.amazonaws.com/6525256482c9e9a06c7a9d3c%2F65b01a129ca976faa7d4d2e3_photo_2023-10-13_14-10-46.webp" alt="Concurrency & Parallelism" width="624" height="354" /> Concurrency & Parallelism <h4>Reliability</h4> Avoidance of side effects and mutable state reduces the risk of bugs and makes code more predictable and maintainable. This is particularly valuable in industries like finance, healthcare, and aerospace, where software reliability is paramount. <h4>Modularity and Reusability</h4> Functional programming encourages the creation of modular and reusable code, enabling developers to build complex systems by composing smaller, well-defined components. This approach reduces development time and promotes code quality. Modularity is enhanced when data is kept immutable because it enforces a clear separation of concerns, predictable behavior, and facilitates the encapsulation of state within modules. Functions that operate on immutable data contribute to this modularity by ensuring that they don't alter the state of the data they work with, making them self-contained and easier to reason about. This design approach leads to more maintainable, testable, and scalable software systems, ultimately saving time and resources. <h4>Declarative Style</h4> The declarative programming style, where you express what you want to achieve rather than how to achieve it, leads to more concise and readable code, simplifying maintenance and collaboration among developers. Organizations can benefit from increased developer productivity and reduced maintenance costs as teams can more easily understand and work with the codebase. <h4>Functional Languages and Libraries</h4> The availability of functional programming languages and libraries, such as R, Haskell, Scala, Clojure, and Elixir, has made it easier for developers to embrace the principles of functional programming. Additionally, major programming languages like JavaScript and Python have incorporated functional features and libraries. This broad ecosystem streamlines development efforts, enabling businesses to leverage existing tools and resources to deliver software efficiently. <img class="size-full wp-image-21199" src="https://webflow-prod-assets.s3.amazonaws.com/6525256482c9e9a06c7a9d3c%2F65b01a13aac7a284a0ecbbbd_photo_2023-10-13_14-10-43.webp" alt="Some Javascript Libraries" width="397" height="397" /> Some Javascript Libraries <h4>Demand for Scalability</h4> In industries like e-commerce and social media, where scaling applications is crucial, its modular and composable nature provides a valuable solution for building robust, scalable systems. This scalability is essential for organizations experiencing rapid growth or fluctuations in user demand, ensuring that their software can adapt and perform reliably under varying conditions. <h4>Community and Education</h4> The community of functional programming has grown significantly, providing resources, tutorials, and a supportive environment for developers looking to adopt these principles. Educational initiatives and online courses have also contributed to the rise in FP adoption. <h2>Conclusion</h2> By elevating the role of functions, emphasizing immutability, and encouraging a declarative style, functional programming offers a versatile framework that addresses the ever-evolving challenges of data science and modern software. As industries and technologies continue to evolve, the principles of functional programming resonate with those seeking innovative solutions. Have questions about functional programming in R or need support with your enterprise R/Shiny project? Make sure to drop us a message. Looking for a way to kickstart your journey? <a href="https://share.hsforms.com/1J9uLL_NdSdW4wADt50b1Vw2rk4g?utm_source=website&utm_medium=blog&utm_campaign=fp1" target="_blank" rel="noopener">Dive into our FREE eBook on Functional Programming in R.</a> <a href="https://share.hsforms.com/1J9uLL_NdSdW4wADt50b1Vw2rk4g?utm_source=website&utm_medium=blog&utm_campaign=fp1" target="_blank" rel="noopener"><img class="aligncenter size-full wp-image-22440" src="https://webflow-prod-assets.s3.amazonaws.com/6525256482c9e9a06c7a9d3c%2F65b019e0fd64fc374268cb9f_Artboard1_5.webp" alt="functional programming ebook" width="1045" height="383" /></a>
Contact us!
